WordPress + VS Code PHP Sniffer & Beautifier
To set up you WordPress project locally to work with solid checks for coding standards there are several ways to do it. You could do checks on the repository post push or merge, you could do it in your workspace project with PHP Code Sniffer and finally you do the package setup globally. If you […]
WP Admin User Addition
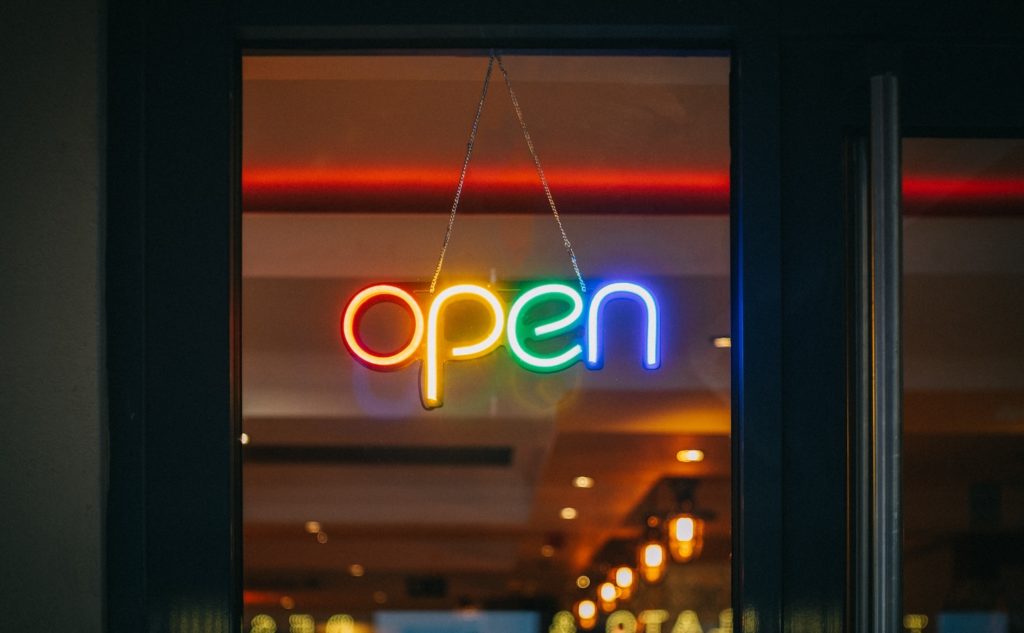
To quickly add a WordPress user to a site to get access there is a hack possible. This by adding code to theme’s function.php using wp_create_user and set_role
Avoiding Extract() in WordPress
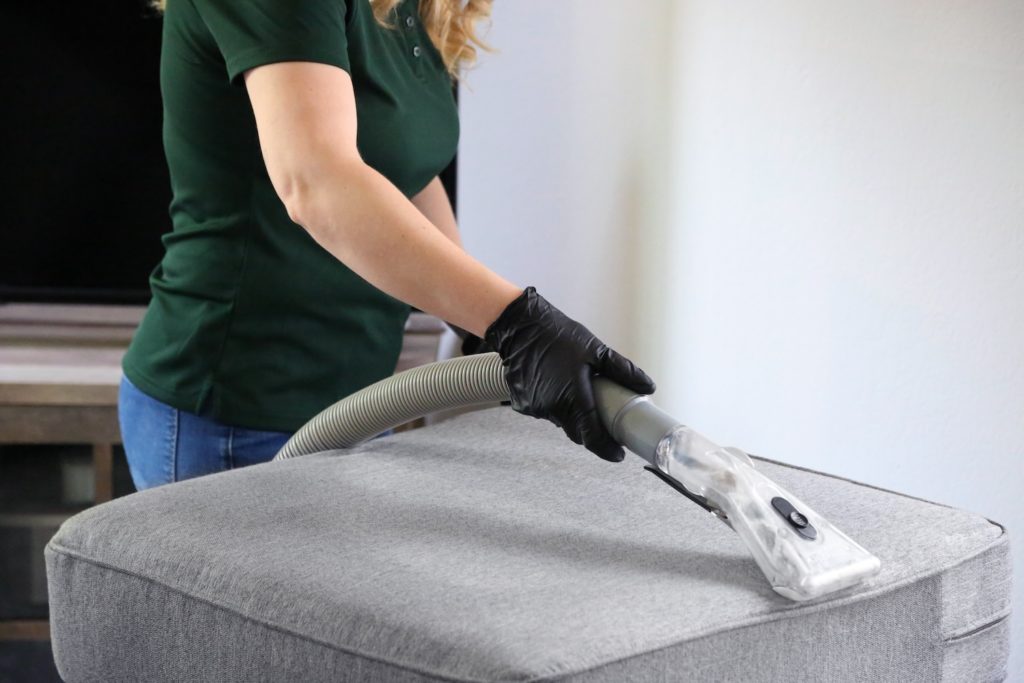
As was decided a long time ago in WordPress extract() should not be used in the case here with shortcode attributes and in general WordPress removed it usage from WordPress core for several reasons. Then there are the times that I can’t find any reference to where a variable came from at all. It’s just […]
Fatal Error Wordfence Test
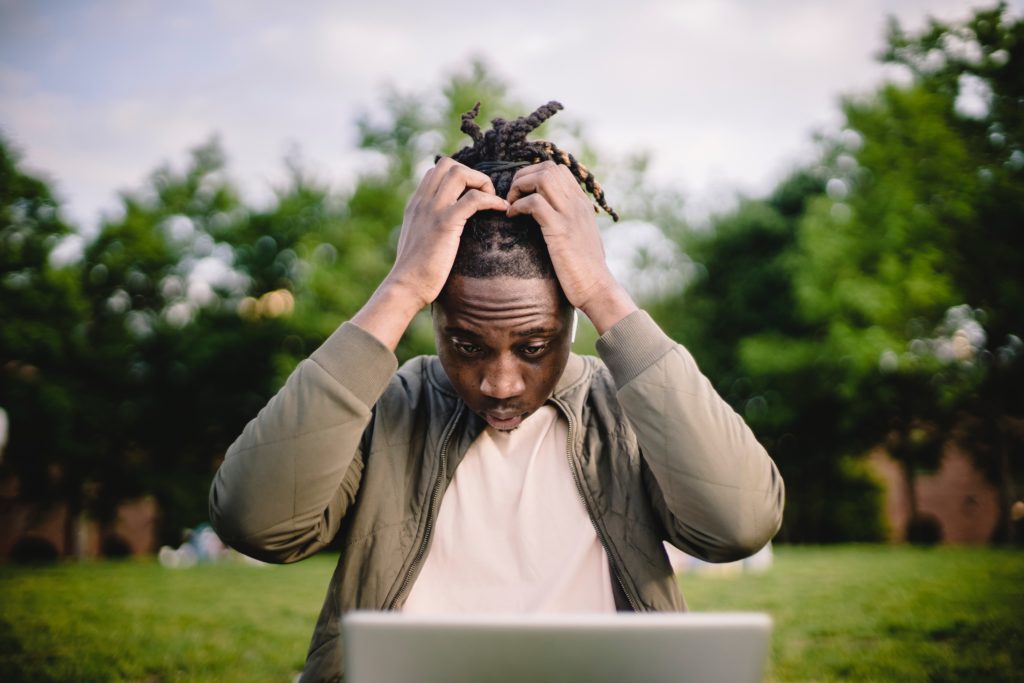
Our customer experienced an error using Amelia Forms. It would not load properly even though we had added its scripts to the exception list in WP Rocket. So we decided to investigate. The only errors in logs we found were from yesterday and were not related to Amelia but to Wordfence. It seemed it did […]
Body Tag User Role
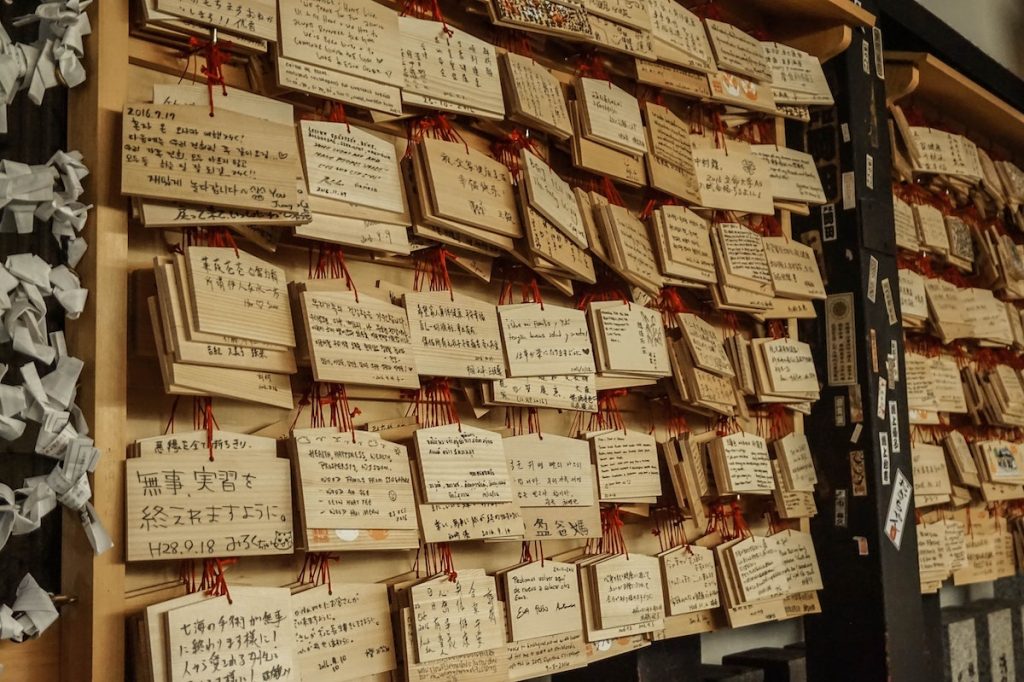
Sometimes you want to add a body tag for a specific user role. This as you want to hide or show specific parts of a page, or site for a particular user. Here is how you can do just that. add_filter( ‘body_class’, ‘imwz_role__body_class’ ); function imwz_role__body_class( $classes ) { $user = wp_get_current_user(); if ( in_array( […]
Assign Specific Role on Registration
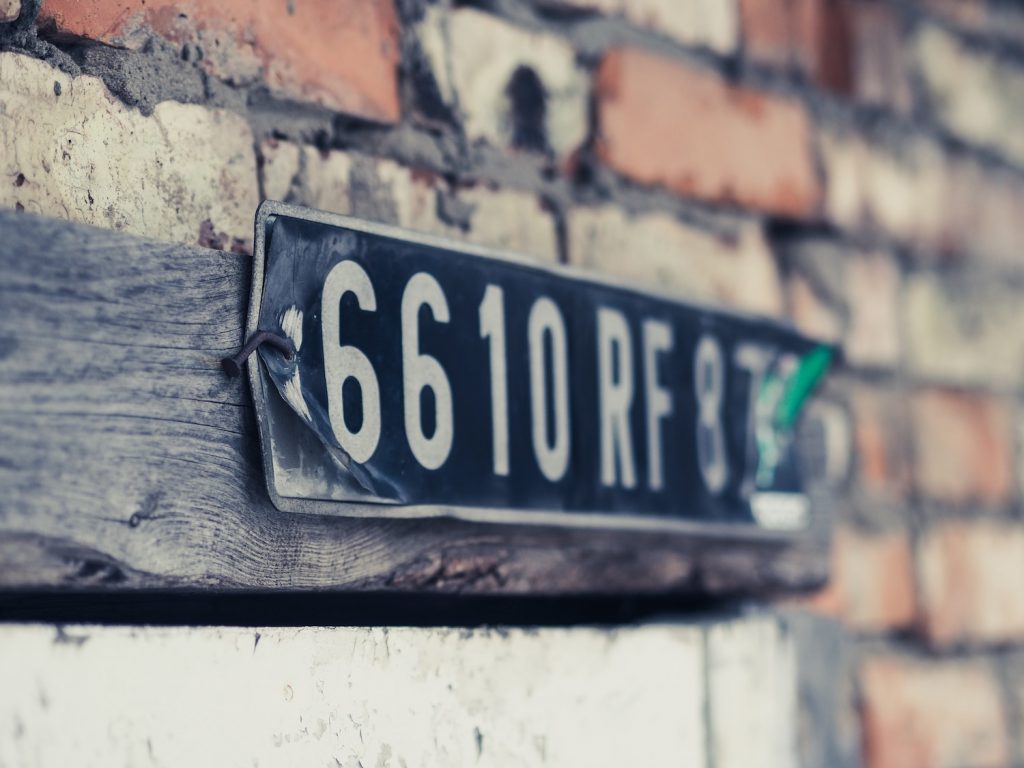
Sometimes you need a custom registration form. A registration form where more fields can be added and where you can assign a specific role based on user input. Plugin Registration Better to put the to be added code in a mu-plugins plugin instead of the theme’s function.php. So let’s start with that. <?php /* Plugin […]
Apply Filter to Specific User
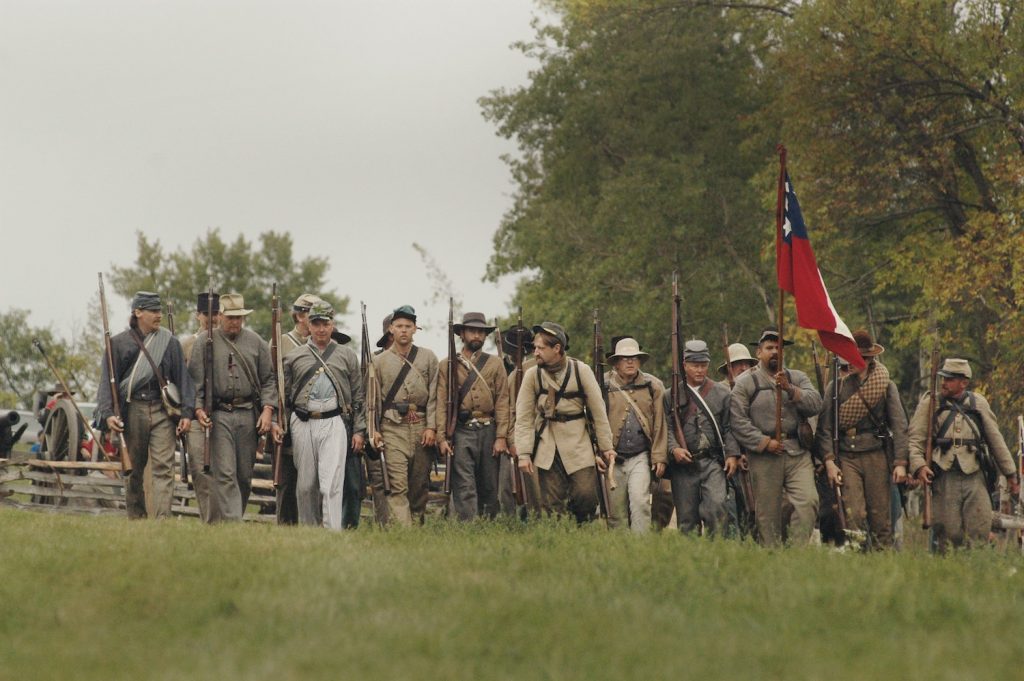
What if you need to apply a certain filter for a certain user? What could you do to achieve this? Well, there is too things we would have to look at. The user we want to work with and the filter to use. User Capabilities Add User Role User Capabilities Check if user has a […]
Redirect Certain Categories to new site
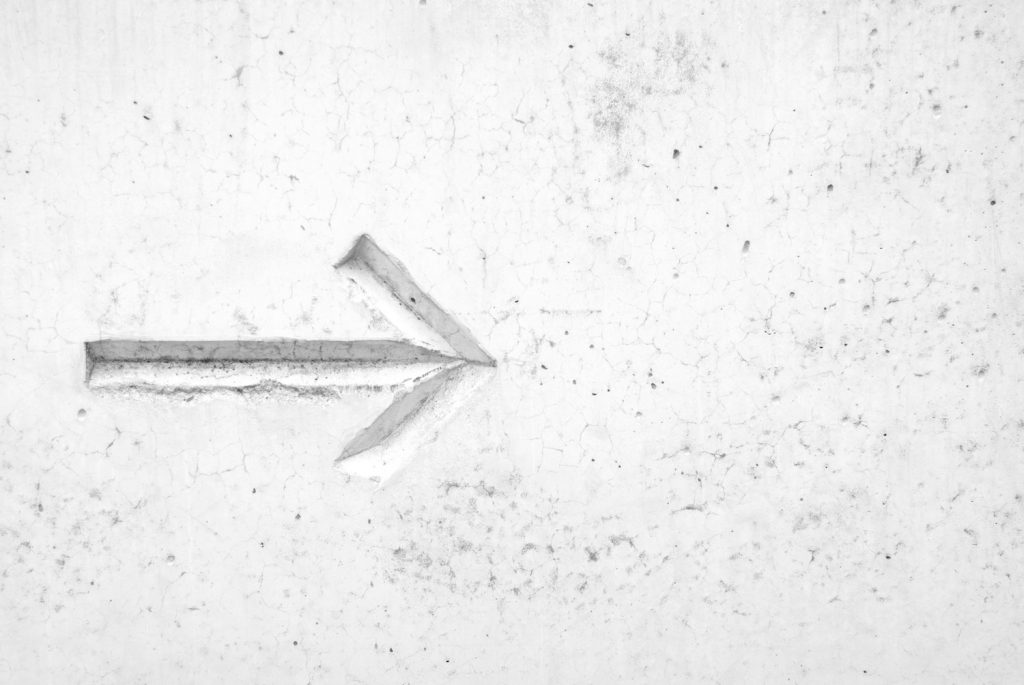
Sometimes you want to redirect posts in certain categories to a new location / domain name. You do not want to redirect the entire website to a new website, but only part of it. This because you perhaps split up your website into multiple websites. Well you are in luck. With WordPress this is possible […]
Block Password Recovery Attacks
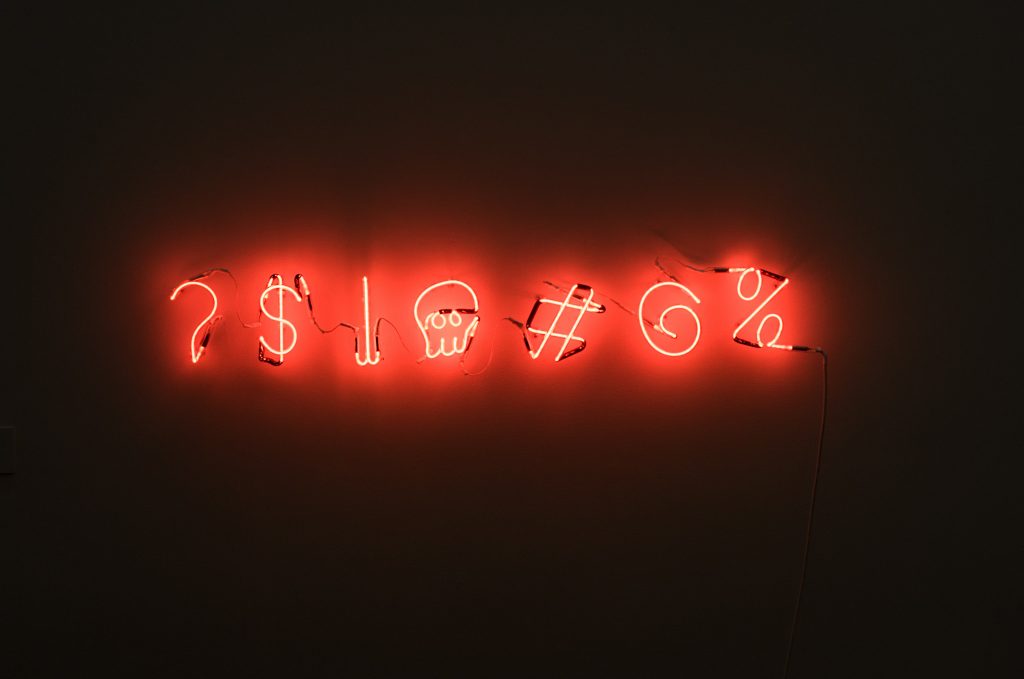
Been annoyed by the recent barrage of brute force password recovery attacks. In WordPress you can block these kind of attacks by turning off password recovery. Now this is obviously not for everyone as you will not be able to reset your password if you ever lose it. And that would mean adjusting the code […]
WordPress Setup Digital Ocean
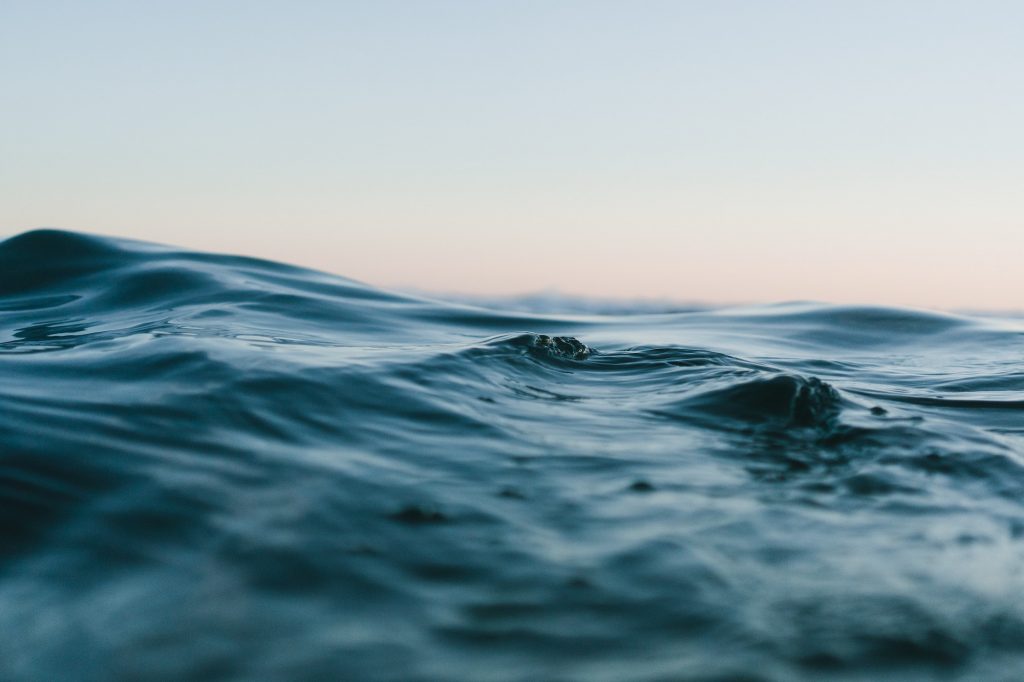
Digital Ocean Account Setup To work with Digital Ocean you need an account. It can be one run by developer, but preferably one client runs and to which he invites you as a developer and or DevOps. So you need the following for starters Setup of account by client with DO Team Setup with access […]